REST API¶
Apptimize provides a REST-based endpoint that allows for the execution of experiments and tracking of user events on any platform.
If you are using Apptimize on a platform that we’ve released a native SDK for, we highly recommend using the SDK which has been optimized for performance and minimal network traffic. However, the REST API provides the flexibility to retrieve and submit Apptimize data from platforms without a native SDK.
Experiments that are executed using the REST API will still need to be created on the Apptimize dashboard, just like experiments on other platforms.
API Endpoints¶
Use the appropriate REST endpoint for your account’s geographic region, either North America or Europe. Our EU cloud site is identical to our NA site in every way, except that all data is handled within the EU, with traffic routed over EU-specific APIs.
- North America base URL
https://api.apptimize.com
- EU base URL
https://api.apptimize.eu
Authentication¶
Authentication is done via an Apptimize API Token, which can be found on the Install page of the Apptimize dashboard. Note that the API Token is different from the App Key used with Apptimize SDKs.
The API Token should be included in the HTTP header as ApptimizeApiToken:
[API Token]
.
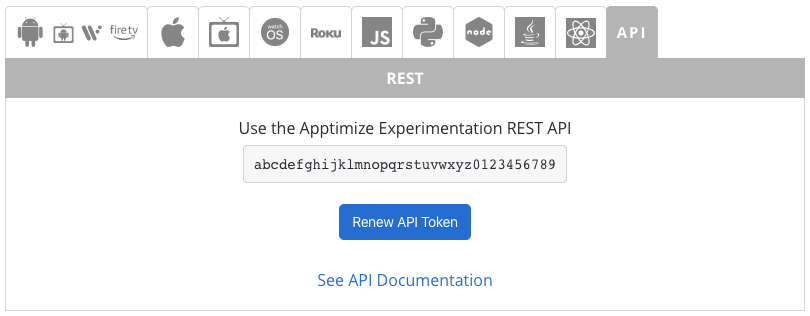
Optional Application Attribute Headers¶
In addition, the headers can include information about your app which can be used for targeting of users in specific experiments or segmentation of results. These include:
Application Attribute |
Required? |
Description |
---|---|---|
ApptimizeApiToken |
Y |
API Token from the Apptimize dashboard |
ApptimizeApplicationName |
N |
Name of the customer application |
ApptimizeApplicationVersion |
N |
Version number of the customer application |
ApptimizeOperatingSystem |
N |
The environment the API is being used in, e.g. “Ruby” |
ApptimizeOperatingSystemVersion |
N |
The environment version the API is being used in |
ApptimizePilotTargetingId |
N |
Pilot Targeting ID for the user |
Mixpanel Support¶
Mixpanel cohort targeting is available through REST. Cohort membership is determined through the user-id
attribute in the Experiments API. The ID is not passed to Mixpanel and can only be used for targeting existing users in cohorts.
For additional details and implementation, please see our Mixpanel Integration
Experiments API¶
The Experiment endpoints provide information on which variant the users have been allocated into specific A/B experiments or feature flags. If the experiment is targeted using either the application attributes above or custom user attributes, make sure you include these optional parameters in each request.
Code Block¶
Retrieve the Code Block variant for a user. Making this call will result in the user being marked as a participant in the given experiment.
GET |
/v1/users/:user-id/code-blocks/:code-block-variable-name |
Parameters
- user-id
String
Customer-provided user ID- code-block-variable-name
String
Name of the code block variable from the Apptimize dashboard- customAttributes
JSON object
(optional) Attributes defined by the user in the form {key:value}, where key is always a string, and value can be any data type supported by JSON.
$ curl -X GET -H "ApptimizeApiToken: <ApiToken>" \
https://api.apptimize.com/v1/users/<user-id>/code-blocks/<code-block-name>
$ curl -X GET -H "ApptimizeApiToken: <ApiToken>" \
-G --data-urlencode "customAttributes={\"<key-1>\":<value-1>,\"<key-2>\":<value-2>}"
https://api.apptimize.com/v1/users/<user-id>/code-blocks/<code-block-name>
{
"codeBlockVariant": "{baseline|variation#}"
}
Dynamic Variable¶
Retrieve the value of a dynamic variable for a user. Making this call will result in the user being marked as a participant in the given experiment.
GET |
/v1/users/:user-id/dynamic-variables/:type/:dynamic-variable-name |
Parameters
- user-id
String
Customer-provided user ID- dynamic-variable-name
String
Name of the dynamic variable from the Apptimize dashboard- type
Enum(String)
Must be one of [bool, bool-array, bool-dictionary, double, double-array, double-dictionary, int, int-array, int-dictionary, string, string-array, string-dictionary]- defaultValue
[Depends on type]
The default value of the dynamic variable if it does not exist – this value must be of the same type as specified in the type parameter.- customAttributes
JSON object
(optional) Attributes defined by the user in the form {key:value}, where key is always a string, and value can be any data type supported by JSON.
$ curl -X GET -H "ApptimizeApiToken: <ApiToken>" \
-G --data-urlencode "defaultValue=\"<default-value>\"" \
https://api.apptimize.com/v1/users/<user-id>/dynamic-variables/string/<dynamic-variable-name>
{
"value": <value>
}
Returned if the provided type is not in the enumeration of dynamic variable
types, or the default value is of a wrong type or is missing.
Note
Use the -G option to pass custom attributes with a GET request.
Feature Flag¶
Retrieve the Feature Flag status (on or off) for a user.
GET |
/v1/users/:user-id/feature-flags/:feature-flag-variable-name |
Parameters
- user-id
String
Customer-provided user ID- feature-flag-variable-name
String
Name of the Feature Flag variable from the Apptimize dashboard- customAttributes
JSON object
(optional) Attributes defined by the user in the form {key:value}, where key is always a string, and value can be any data type supported by JSON.
$ curl -X GET -H "ApptimizeApiToken: <ApiToken>" \
https://api.apptimize.com/v1/users/<user-id>/feature-flags/<feature-flag-variable>
$ curl -X GET -H "ApptimizeApiToken: <ApiToken>" \
-G --data-urlencode "customAttributes={\"<key-1>\":<value-1>,\"<key-2>\":<value-2>}" \
https://api.apptimize.com/v1/users/<user-id>/feature-flags/<feature-flag-variable>
{
"isFeatureFlagEnabled":{true|false}
}
Variant Info¶
Retrieve detailed information about the experiments a given user is enrolled in.
GET |
/v1/users/:user-id/variant-info |
Parameters
- user-id
String
Customer-provided user ID- customAttributes
JSON object
(optional) Attributes associated with the user
$ curl -X GET -H "ApptimizeApiToken: <ApiToken>" \
https://api.apptimize.com/v1/users/<user-id>/variant-info
{
"variants": [list of variant objects]
}
{
"variantName": "<string>",
"variantID": <number>,
"experimentName": "<string>",
"experimentType": "<string>",
"experimentID": <number>,
"cycle": <number>,
"currentPhase": <number>
}
Events API¶
The Events endpoint allows you to providing tracking data for a given user. These events can then be used as goals in order to measure the success of an experiment. Events tracked through the REST API will be available as goals for experiments that are executed on any platform, not just those that are executed using the REST API.
Track an Event¶
Log an event associated with a user.
POST |
/v1/users/:user-id/events/:event-name |
Parameters
- user-id
String
Customer-provided user ID- event-name
String
Name of the event to track- value
Number
(optional, body) Value associated with the event (e.g. revenue)- customAttributes
JSON object
(optional, body) Attributes associated with the user
Note
Use the -F option to pass custom attributes as form fields with a POST request
$ curl -X POST -H "ApptimizeApiToken: <ApiToken>" -F "value=<double value>" \
https://api.apptimize.com/v1/users/<user-id>/events/<event-name>
No Content
See also
curl
documentation